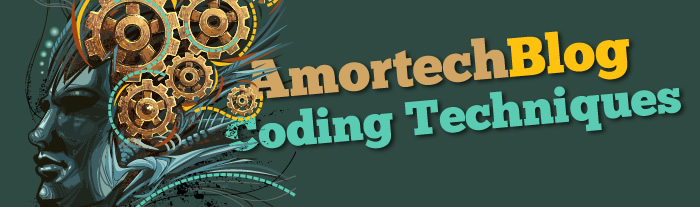
Here some great shorthand techniques that are valuable for any Javascript based developer. These will save both time and space – keeping your code clean and whitespace to a minimum.
1. Shorthand – Null, Undefined, “” Checks
A common scenerio for developers is to check if the variable you are referencing is null or undefined.
Longhand:
if (variable1 !== null || variable1 !== undefined || variable1 !== '') {
var variable2 = variable1;
}
Shorthand:
var variable2 = variable1 || '';
2. Shorthand – If true … else
This is based on the old school C++ shorthand and actually applies to many languages
Longhand:
var big; if (x > 10) { big = true; } else { big = false; }
Shorthand:
var big = (x > 10) ? true : false;
3. Shorthand – Associative Array Notation
Back in the day we would create a names array and then add each named element. Now we can use object literal notation which is quicker and more readable.
Longhand:
var physicalFeatures = new Array(); physicalFeatures['Hair Color'] = 'Black'; physicalFeatures['Eye Color'] = 'Blue'; physicalFeatures['Hair Length'] = 'Long'; physicalFeatures['Hair Style'] = 'Curly';
Shorthand:
var physicalFeatures = { 'Hair Color' : 'Black', 'Eye Color' : 'Blue', 'Hair Length' : 'Long', 'Hair Style' : 'Curly' };
3. Shorthand – Object Array Notation
Want to declare an array in one line? Here ya go:
Longhand:
var a = new Array(); a[0] = "string1"; a[1] = "string2"; a[2] = "string3";
Shorthand:
var a = ["string1", "string2", "string3"];
5. Shorthand – Assignment Operators
Some some simplified arithmetic operations.
Longhand:
x=x+1; minusCount = minusCount - 1; y=y*10;
Shorthand:
x++; minusCount --; y*=10;
Other helpful operators given that x=10 and y=5:
x += y //result x=15 x -= y //result x=5 x *= y //result x=50 x /= y //result x=2 x %= y //result x=0
6. Shorthand – charAt()
This function is used to find a character at a certain position.
Longhand:
"string".charAt(0);
Shorthand:
"string"[0]; // Returns 's'
7. Shorthand – If Presence
This should be a well known shorthand but it’s worht mentioning:
Longhand:
if (likeJavaScript == true)
Shorthand:
if (likeJavaScript)
Longhand:
var a; if ( a != true ) { // do something... }
Shorthand:
var a; if ( !a ) { // do something... }
8. Shorthand – Regex Object
This is the proper way to declare a regex object if you are planning to reuse the object.
Longhand:
searchText = "padding 1234 riverbend drive calgary ab more padding" /d+.+n{0,2}.+s+[A-Z]{2}s+d{5}/m(searchText) //returns: ["1234 riverbend drive calgary ab"]
Shorthand:
var re = new RegExp(/d+.+n{0,2}.+s+[A-Z]{2}s+d{5}/m); re.exec(searchText); //returns: ["1234 riverbend drive calgary ab"]
9. Shorthand – foreach Loop
The jQuery.each function does a much better job but if you are limited to only javascript here is a nice shorthand solution.
Longhand:
for (var i = 0; i < array.length; i++)
Shorthand:
for(var i in array)
Conclusion
Like these shorthands or find them useful? Please comment. Did we miss any handy ones? Please free to leave yours below.
Amortech Design Labs specializes in creative web development in the Calgary area. We support clean and manageable code giving you extreme value and reusability.
Recent Comments